- OctoMYQtDepends: Our helper to include dependency on all the Qt modules that OctoMY™ needs
- OctoMYLibProbe: Our helper to enumeratelibrary folders
- OctoMYFiles: Our helper to list source files in a project that we care about (this is equivalent to tile fil Group in our StaticLibrary above).
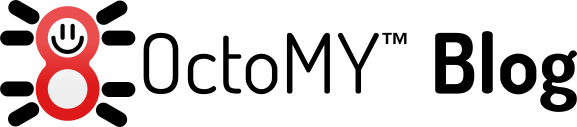
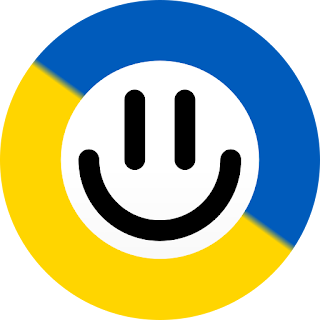
This is the official blog about the development of OctoMY™, the robot platform for you!
2023-02-24
Qbs tutorial part 5/5: Apps
Qbs tutorial part 4/5: File Groups
When specifying files, you can use the wildcards "*", "?" and "[]", which have their usual meaning. By default, matching files are only picked up directly from the parent directory, but you can tell Qbs to consider the whole directory tree. It is also possible to exclude certain files from the list. The pattern ** used in a pathname expansion context will match all files and zero or more directories and subdirectories.
Qbs tutorial part 3/5: Libraries
Qbs tutorial part 2/5: Tests
- srcDir
- projectDir
- testDir
- commonTestDir
- commonTestLib
- testNames - a list of the names of tests found in our project
- testFolders - the folders for each of our tests
- testFiles - the test.qbs file for each test
- testDefines - some defines that refer to our test names
Qbs tutorial part 1/5: Main project file
After spending a few gruelling weeks porting my brain to "think in Qbs", I felt ready to take on the non-trivial task of comitting 100% to porting the build system of OctoMY™ from qmake to Qbs. This was in large part possible thanks to the super-human patience and wisdom demonstrated by the super awesome Qbs community, which lives on Discord. Shoutout to ABBAPOH, Psy-Kai and Janet Blackquill, your help was indispensible!
I thought I would document my decisions here for future reference and maybe to serve as a template for others trying to get into Qbs with their non-trivial projects. I will also introduce some concepts and tips & tricks that I picked up along the way.
So first up: top level project structure. OctoMY™ is divided coarsely into 3 parts:
- Apps - The actual programs that we run when we want to use OctoMY™
- Libs - The software components which contains the code of OctoMY™, liked into the apps
- Tests - A bunch of programs, each testing one aspect of the codebase
- import qbs.FileInfo: This is how we get access to other types and script libraries in qbs. In this case, we need the FileInfo service provided with qbs that allows us to do file operations.
- Project: This indicates that we are defining a new qbs project. "Project" is a qbs type that represents a project. It is used to collect other items inside of it similar to a folder in a filesystem. It can contain other items directly inside of it, or it can refer to files which in turn will contain such items. In our case we only refer to other files through the references property (see below).
- name: This is what shows up inside QtCreator as the name of your project. If you leave it out, Qbs will use the file as a fallback. In other words, this property is optional.
- qbsSearchPaths: This points to where qbs will look for our user defined reusable components, of which there are several in the OctoMY™ project. You don't have to use this feature, but it will serve you well to use it once your Qbs project grows beyond the trivial "Hello World" examples. In this case we tell qbs that this project will look for our components in ./integrations/qbs folder, and you can see (in theproject tree at the top of this article) that it contains some components that we will use from sub projects, such as OctoMYTestProbe.qbs.
- references: References is a list of files that Qbs should read and include in the project. Since we are currently defining a project, we want to include the actual sources and libraries and tests of the project, and that is done by referring to the qbs files which we use to find them. In this case we include 3 sub-projects via the libs.qbs, apps.qbs and test.qbs files.
OctoMY™ will migrate from qmake to Qbs
OctoMY™ has from this date forward decided to standardize on Qbs and migrate from qmake to Qbs. qmake project files will remain for some time until we see that the Qbs project files replace them perfectly before they will be removed. Thanks for all the fish qmake!
This might seem like a strange decision since Qt itself has selected CMake in favor of their own Qbs project, however the benefits Qbs will have to OctoMY™ is substancial compared to what CMake will bring. So here is the list of reasons why OctoMY™ will chose Qbs over CMake:
- CMake is arguably hard to work with, inelegant, cargo-culty, full of legacy, slow, inflexible, archaic and challenging to like
- The main selling point of CMake is that "everyone is using it" and so you will find that a lot of projects support CMake and consuming these projects as dependencies with CMake will be simpler. This is however not relevant for OctoMY™ because we have a strict policy of not depending on any external libraries except Qt framework itself. All the sources are in the tree.
- CMake will allow us to export our project as a dependency to others. Again, this is a mute point as OctoMY™ will not be consumed as a source level dependency.
- Qbs is well supported by Qt (they developed it after all)
- Qbs is well integrated into QtCreator which is very important for OctoMY™ since we depend on that as our main development tool
- Qbs is modern
- Qbs is flexible
- Qbs is fast
- Qbs is multi-platform and has good support for all the platforms that OctoMY™ do and will need support for in the future
- Qbs is open-source
- Qbs is well written (if you peek inside the sources of Qbs, you will probably like what you see)
- Qbs acts as a server that talks to QtCreator over a pipe. This means that QtCreator will better reflect the internal state of Qbs at any given time. For example, it would automatically update the project tree view in QtCreator whenever I saved changes to the project (*.qbs) files, and Qbs would act as expected in many cases where qmake just would not. This gave a surprisingly fluid experience that is addictive.
- Qbs really maxes out your CPU at build time and spends almost zero time on house-keeping. The system is really fast at dependency checking and re-building only the necessary files making your developer experience quite pleasant.
- It has not yet screwed up dependencies even once even in my very demanding project and with me as a demanding user which is quite impressive. qmake would require a full re-build on a regular basis.
- While Qbs works out of the box with Qt, it is not limited to Qt. It has extensions to support a whole load of platforms, languages and frameworks and can integrate with a bunch of developer tools including IDEs, other build tools and more. Especially, Qbs aims to tackle the not-for-the-faint-of-heart builds that target embedded hardware, mobile platforms and other quirky and archaic targets full of demanding requirements such as cross compilation and advanced tool-chain management. Impressive! This means we have some wiggle-room for futer expansion of the OctoMY™ project
- There is a Qbs Discord Server where devs and users of Qbs hang out. This has been one of the best discoveries so far. The amount of patience and wisdom the core team have showed me as a annoying beginner is truely amazing.
- Qbs will hopefully grow and get a much deserved boost in adoption!
- The Qbs output is very sparse and debugging Qbs is very difficult for a beginner in the start before you start udnerstanding how it all works under the hood. The log output windows in QtCreator will all just be completely empty even if there definitely are some problems. There are options to add more verbose output, but it is well hidden and off by default. Also the "max verbose" option is not possible to swithc on at all.
- "printf debugging" is hard since Qbs is declarative. It is definitely possible but very quirky.
- Some of the features in Qbs have severe pitfalls that you are expected to pick up on by yourself. Here are some examples:
- Group prefix is a string, and NOT a file entity. In other words, "someFolder" and "someFolder/" means different things.
- While the documentation is "complete" it is kind of sparse still, and leaves you wondering about implementation details in many cases. For example, does Group.excludeFiles support wildcards like Group.files or not? (The answer is yes, it does, but the documentation does not mention this).
2023-02-07
Old dog learns new tricks: Qbs edition
So I had a go at QML a few years ago and it seems like a cool technology and definitely has a lot of potential for creating good user interfaces.
Getting started with QML is easy enough thanks to the examples directly present in QtCreator. You can get up and running quickly and adapt an example to what you want to achieve.
After a while you will stumble upon a bunch of more or less fundamental questions like:
- How can I interface QML with my existing C++ code?
- How can I get the filename and path of the current module from code?
- What is the meaning of life?
2023-02-02
Brief OctoMY™ update 2023
These are the changes scheduled for early to mid 2023 in the OctoMY™ project:
- Good bye Qt5 hello Qt6
- Refactoring of subsystems relating to media such as speech, video streaming etc
- Once-over of whole project
- New build system